Java界面实现的简单绘图板
创建界面与面板
创建界面与实现监听的具体方式笔者已经在之前的博客中进行了详细的介绍,这里就不再赘述。
首先我们创建一个界面,并在界面中设置面板区域:
1 | JFrame jf=new JFrame(); |
在这几行代码中,我们为每个面板规定了大小和背景色。但要注意的有:
南北(上下)方向的面板宽度规定为整个界面,东西(左右)方向的面板高度规定为顶格至南北方向面板,所以我们无需再考虑已经规定的长度,写成0即可。
中央的面板的大小在其余四个方向面板规定后就无需设置,当然,设置了也没有问题,但要注意大小,不然可能会有“画出界”的情况发生。
设置的背景色可以用color(R,G,B)来实现,用一般电脑自带的画图软件就能够获取心仪颜色的参数:
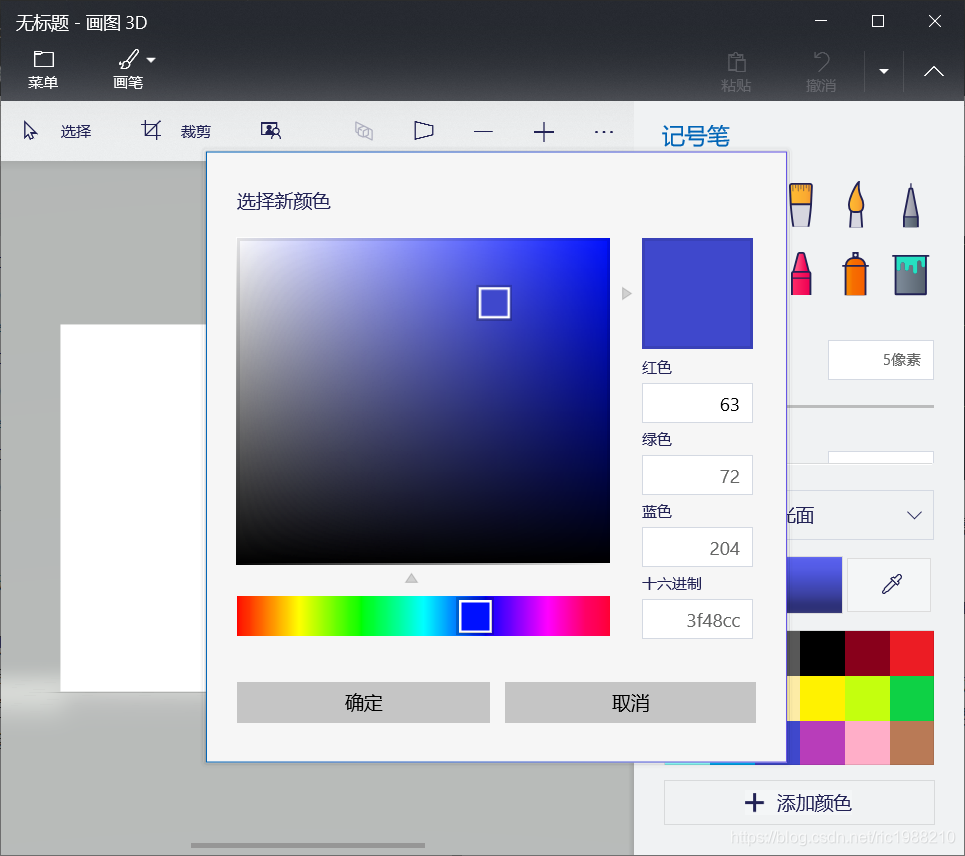
记得将每个面板添加到界面中jf.add(jpn,BorderLayout.NORTH); ......jf.add(jpc,BorderLayout.CENTER);
并设置其添加方向。
这样一个画图板界面就初步实现了:
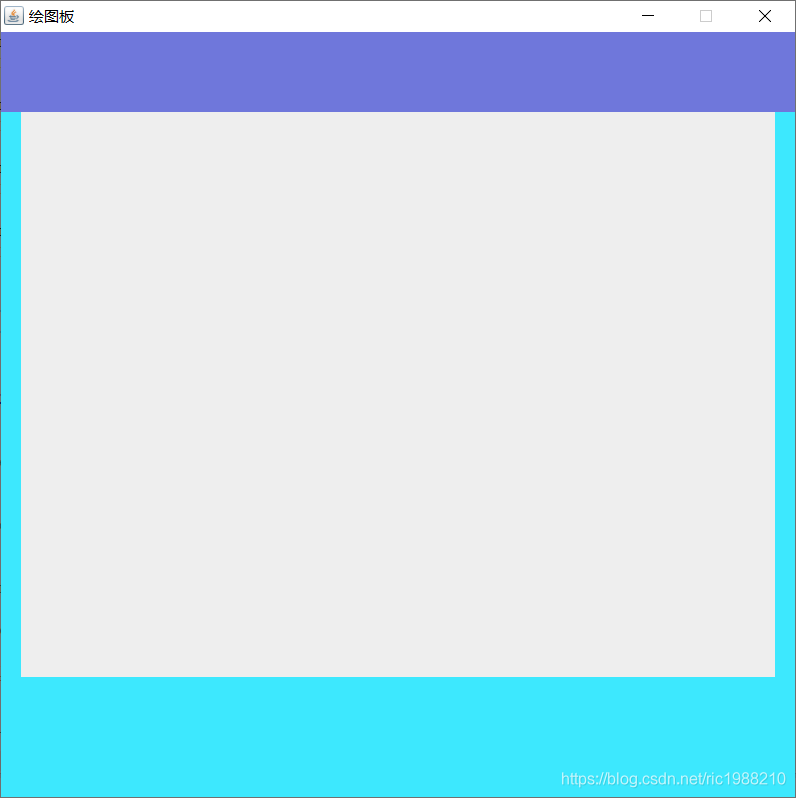
1 | Color[] btnColors = {Color.red,Color.blue,Color.orange,Color.yellow,Color.black}; |
1 | JButton ccl=new JButton("自选色"); |
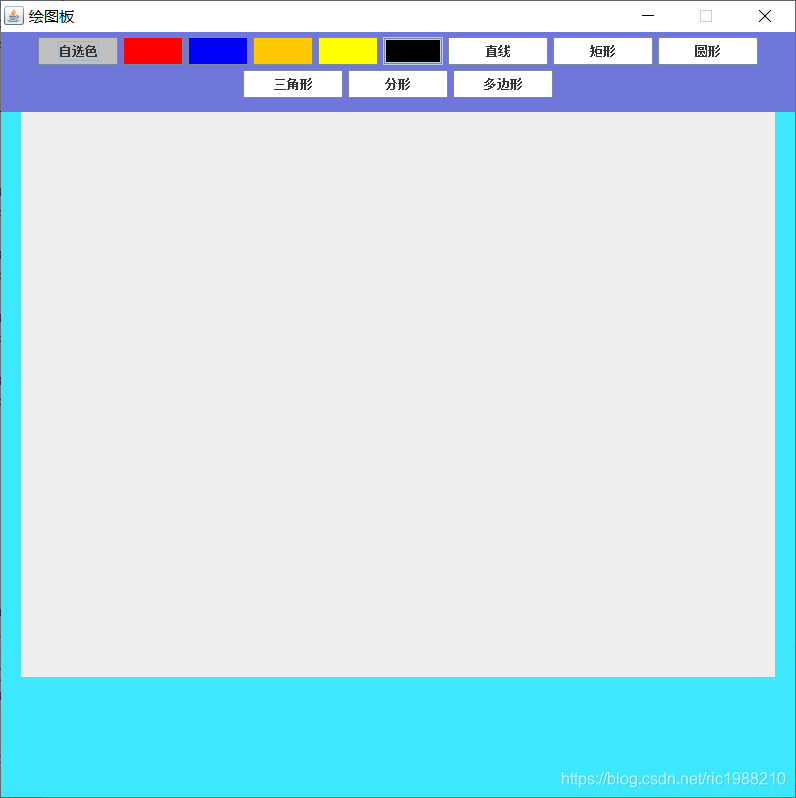
画图动作的监听与实现
为了实现一个画图板,我们需要给监听器引用两个接口,即 MouseListener和ActionListener。我们先定义变量和抽象方法:
1 | int x1, x2, y1, y2, x3, y3, x4, y4, x5, y5; |
之后我们重载这些方法:
1 | public void actionPerformed(ActionEvent e) { |
这其中鼠标监听器中的方法大家可以自行查看,具体方法为高亮MouseListener
后同时按下Ctrl+鼠标左键。这其中有几点要注意:
注意点的坐标,选择恰当绘图的起点,不然画出来的图形会“乱跑”。
if (sbtn.equals("自选色")){ Color c2 = JColorChooser.showDialog(null, "颜色提取器", Color.LIGHT_GRAY); graw.setColor(c2); JButton ccl=(JButton) e.getSource(); ccl.setBackground(c2); }
最后,我们将图像绘制在CENTER面板中,这样我们就可以用它来画一些简易的图象了! <img src="https://raw.githubusercontent.com/EDAttlee/edattlee.github.io/main/downloaded_images/20191023194128824.png" alt="在这里插入图片描述" style="zoom:67%">1
2
3
4
5
6
7
8
我们引用了Java的颜色提取器,它会在我们选定颜色后将按钮染成我们选定的颜色。注意,JColorChooser.showDialog(Component,String, Color— initialColor)的返回值为颜色,我们将其赋给变量C2。
```java
DrawListener drl=new DrawListener();
jpc.addMouseListener(drl);
......
Graphics g=jpc.getGraphics();
drl.graw=g;